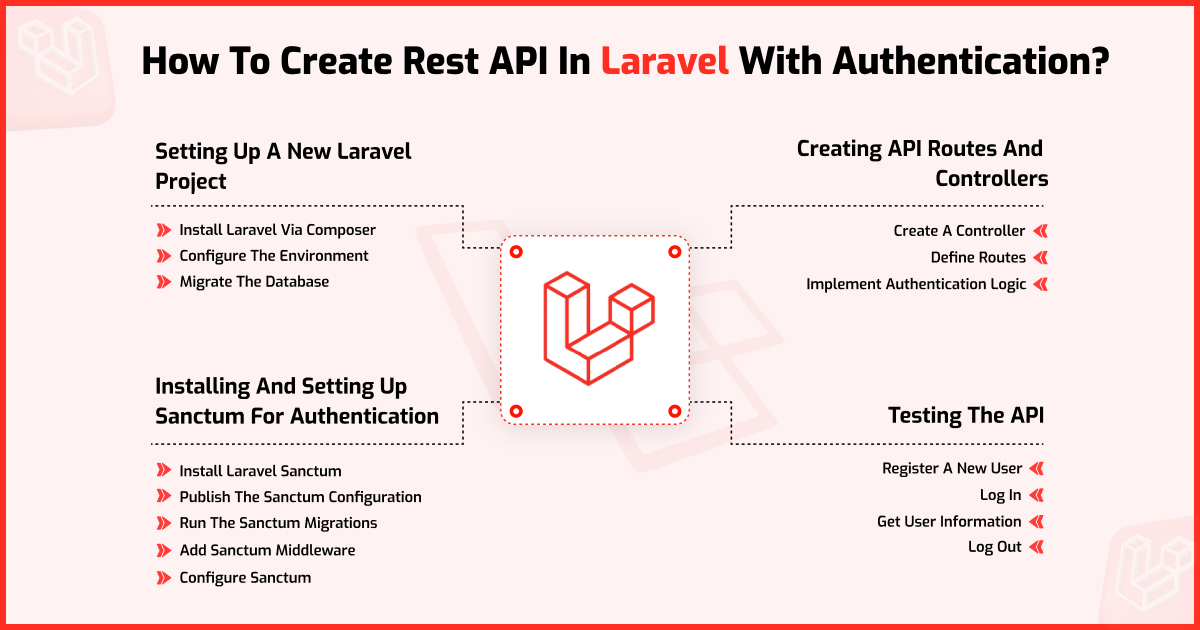
Table of Contents
How to Setup Laravel Login Authentication in 2024 : Certainly! Here’s a full-length, comprehensive guide on Laravel authentication methods, covering password-based, token-based, and multi-factor authentication in detail. It will also include practical steps and examples to enhance understanding and implementation.
How to Setup Laravel Login Authentication
Comprehensive Guide to Laravel Authentication Methods: Password, Token-Based, and Multi-Factor Authentication
Authentication is a fundamental aspect of web development. It ensures that users accessing the system are who they claim to be and secures sensitive data from unauthorized access. Laravel, a versatile and widely-used PHP framework, offers several methods for user authentication. From traditional password-based authentication to more advanced token-based and multi-factor authentication, Laravel provides robust and easily implementable solutions for developers.
In this detailed guide, we will explore some of the most commonly used Laravel authentication methods:
- Password-Based Authentication
- Token-Based Authentication
- Multi-Factor Authentication (MFA)
How to Setup Laravel Login Authentication
Password-Based Authentication in Laravel: A Comprehensive Guide
Password-based authentication is the most common form of authentication in web applications, where users are required to log in using a unique identifier (like an email or username) along with a password. Laravel, being a comprehensive and developer-friendly framework, provides a built-in system to easily manage password-based authentication.
In this section, we’ll delve into the step-by-step process of setting up password-based authentication in Laravel, covering everything from configuring routes to managing password resets and securing user credentials.
How to Setup Laravel Login Authentication
1. Introduction to Password-Based Authentication
Password-based authentication is a straightforward method that checks user credentials (usually email/username and password) against stored data in the database. Once authenticated, the user is granted access to the system and a session is maintained to track their activity.
Laravel’s built-in authentication system simplifies the process of user registration, login, and password management by handling the backend logic, session management, password hashing, and more.
2. Setting Up Password-Based Authentication in Laravel
Let’s break down the process of setting up password-based authentication in Laravel.
How to Setup Laravel Login Authentication
Step 1: Installing Laravel 9
Before setting up password-based authentication, ensure that you have Laravel installed in your environment. If Laravel isn’t installed yet, you can install it using Composer:
composer create-project --prefer-dist laravel/laravel blog
Navigate to the project directory:
cd blog
Step 2: Configuring the Database
For password-based authentication, user credentials need to be stored in a database. Begin by configuring your database connection in the .env
file located in the root directory. Edit the file with the correct database credentials:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_auth
DB_USERNAME=root
DB_PASSWORD=your_password
How to Setup Laravel Login Authentication
After configuring the database, run the following command to create the required tables:
php artisan migrate
This command will create several tables, including the users
table which is essential for managing user data.
Step 3: Installing Laravel Breeze or Jetstream (Optional)
Laravel Breeze and Jetstream are packages that provide an out-of-the-box authentication system, including login, registration, password resets, and more. Breeze is a lightweight option, while Jetstream is more feature-rich.
To install Laravel Breeze:
composer require laravel/breeze --dev
How to Setup Laravel Login Authentication
Once installed, run the following commands:
php artisan breeze:install
npm install && npm run dev
php artisan migrate
Laravel will automatically generate the authentication controllers, routes, and views for you. You can skip this step if you prefer to manually set up authentication.
Step 4: Creating the User Model
If you haven’t used Breeze or Jetstream, you need to manually create a model and migration for users. Laravel already includes a User
model, but you can create one if necessary using:
php artisan make:model User -m
How to Setup Laravel Login Authentication
Update the generated migration file (in database/migrations
) to include fields like name, email, and password:
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
Run the migration:
php artisan migrate
Now, your users table is ready.
Step 5: Setting Up Routes for Authentication
Laravel uses Auth
facade to handle routes related to login, registration, and password management. If you want to manually create authentication routes, add the following to routes/web.php
:
How to Setup Laravel Login Authentication
use App\Http\Controllers\Auth\LoginController;
use App\Http\Controllers\Auth\RegisterController;
Route::get('login', [LoginController::class, 'showLoginForm'])->name('login');
Route::post('login', [LoginController::class, 'login']);
Route::post('logout', [LoginController::class, 'logout'])->name('logout');
Route::get('register', [RegisterController::class, 'showRegistrationForm'])->name('register');
Route::post('register', [RegisterController::class, 'register']);
These routes will direct users to the login and registration forms and handle the authentication process.
If you have installed Laravel Breeze or Jetstream, the authentication routes will already be preconfigured.
Step 6: Creating Controllers for Authentication
Laravel’s authentication logic resides in Auth
controllers. If you haven’t installed Breeze or Jetstream, you’ll need to create the LoginController
and RegisterController
.
To create a login controller:
How to Setup Laravel Login Authentication
php artisan make:controller Auth/LoginController
In this controller, you will handle the logic for authenticating users. A basic login method might look like this:
namespace App\Http\Controllers\Auth;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
class LoginController extends Controller
{
public function showLoginForm()
{
return view('auth.login');
}
public function login(Request $request)
{
$credentials = $request->only('email', 'password');
if (Auth::attempt($credentials)) {
return redirect()->intended('dashboard');
}
return redirect()->back()->withErrors(['error' => 'Invalid credentials']);
}
public function logout()
{
Auth::logout();
return redirect('/');
}
}
Similarly, you can create a RegisterController
for managing user registration.
Step 7: Creating Views for Authentication
Next, you’ll need to create views for login and registration forms. In the resources/views
directory, create a new folder named auth
and add login.blade.php
and register.blade.php
files.
For example, here’s a simple login view (login.blade.php
):
How to Setup Laravel Login Authentication
<form method="POST" action="{{ route('login') }}">
@csrf
<div>
<label for="email">Email:</label>
<input type="email" name="email" required autofocus>
</div>
<div>
<label for="password">Password:</label>
<input type="password" name="password" required>
</div>
<button type="submit">Login</button>
</form>
And a basic registration form (register.blade.php
):
<form method="POST" action="{{ route('register') }}">
@csrf
<div>
<label for="name">Name:</label>
<input type="text" name="name" required autofocus>
</div>
<div>
<label for="email">Email:</label>
<input type="email" name="email" required>
</div>
<div>
<label for="password">Password:</label>
<input type="password" name="password" required>
</div>
<div>
<label for="password_confirmation">Confirm Password:</label>
<input type="password" name="password_confirmation" required>
</div>
<button type="submit">Register</button>
</form>
These views will render simple forms that submit data to the appropriate authentication routes.
Step 8: Securing Passwords with Hashing
When storing user passwords, security is paramount. Laravel makes it easy to hash passwords using the bcrypt
function. You can hash passwords in the RegisterController
before saving them to the database:
How to Setup Laravel Login Authentication
use Illuminate\Support\Facades\Hash;
class RegisterController extends Controller
{
public function register(Request $request)
{
$validatedData = $request->validate([
'name' => 'required|string|max:255',
'email' => 'required|string|email|max:255|unique:users',
'password' => 'required|string|min:8|confirmed',
]);
$user = new User();
$user->name = $validatedData['name'];
$user->email = $validatedData['email'];
$user->password = Hash::make($validatedData['password']);
$user->save();
Auth::login($user);
return redirect()->intended('dashboard');
}
}
This ensures that passwords are securely hashed before being stored in the database.
Step 9: Implementing Password Reset Functionality
Password reset functionality allows users to reset their password via email if they forget it. Laravel’s built-in password reset system handles this with ease. You can add password reset routes to routes/web.php
:
How to Setup Laravel Login Authentication
Route::get('password/reset', [ForgotPasswordController::class, 'showLinkRequestForm'])->name('password.request');
Route::post('password/email', [ForgotPasswordController::class, 'sendResetLinkEmail'])->name('password.email');
Route::get('password/reset/{token}', [ResetPasswordController::class, 'showResetForm'])->name('password.reset');
Route::post('password/reset', [ResetPasswordController::class, 'reset']);
Laravel will handle sending password reset links and resetting user passwords, ensuring that the system is both secure and user-friendly.
3. Best Practices for Password-Based Authentication
To ensure that your password-based authentication system is secure and efficient, follow these best practices:
- Use Strong Password Policies: Enforce strong password policies with a minimum length, special characters, and no common passwords.
- Enable Rate Limiting: Use Laravel’s rate limiting features to prevent brute-force attacks by limiting login attempts.
- Use HTTPS: Always serve your application over HTTPS to prevent man-in-the-middle attacks when transmitting sensitive data like passwords.
- Use Password Hashing: Laravel automatically hashes passwords using the
bcrypt
algorithm. Never store passwords in plain text.
How to Setup Laravel Login Authentication
Token-Based Authentication laravel
Token-Based Authentication in Laravel involves using tokens to authenticate users instead of traditional session-based methods. Laravel provides a built-in package called Laravel Passport for API authentication, but you can also use Laravel Sanctum for lightweight API authentication. Below is a guide to implement Token-Based Authentication using Laravel Sanctum:
Step-by-Step Guide to Implement Token-Based Authentication with Laravel Sanctum
1. Install Laravel Sanctum
First, you need to install Sanctum into your Laravel project. Run the following command in your project’s root directory:
How to Setup Laravel Login Authentication
composer require laravel/sanctum
Next, publish the Sanctum configuration file:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
Run the migrations:
php artisan migrate
Step 2: Configure Sanctum
In your config/auth.php
file, update the api
guard to use Sanctum:
How to Setup Laravel Login Authentication
'guards' => [
'api' => [
'driver' => 'sanctum',
'provider' => 'users',
],
],
Step 3: Set Up Middleware
Add Sanctum’s middleware to your api
middleware group within your app/Http/Kernel.php
file:
'api' => [
\Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class,
'throttle:api',
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
Step 4: Setting Up API Routes
Define the routes for authentication in the routes/api.php
file:
How to Setup Laravel Login Authentication
use App\Http\Controllers\AuthController;
Route::post('/register', [AuthController::class, 'register']);
Route::post('/login', [AuthController::class, 'login']);
Route::middleware('auth:sanctum')->get('/user', [AuthController::class, 'user']);
Route::middleware('auth:sanctum')->post('/logout', [AuthController::class, 'logout']);
Step 5: Create the AuthController
Create a controller for handling authentication:
php artisan make:controller AuthController
In the AuthController.php
, implement methods for registering, logging in, fetching user details, and logging out:
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Auth;
class AuthController extends Controller
{
public function register(Request $request)
{
$request->validate([
'name' => 'required|string|max:255',
'email' => 'required|string|email|max:255|unique:users',
'password' => 'required|string|min:8',
]);
$user = User::create([
'name' => $request->name,
'email' => $request->email,
'password' => Hash::make($request->password),
]);
$token = $user->createToken('auth_token')->plainTextToken;
return response()->json([
'access_token' => $token,
'token_type' => 'Bearer',
]);
}
public function login(Request $request)
{
$request->validate([
'email' => 'required|string|email',
'password' => 'required|string',
]);
if (!Auth::attempt($request->only('email', 'password'))) {
return response()->json(['message' => 'Unauthorized'], 401);
}
$user = User::where('email', $request->email)->firstOrFail();
$token = $user->createToken('auth_token')->plainTextToken;
return response()->json([
'access_token' => $token,
'token_type' => 'Bearer',
]);
}
public function user(Request $request)
{
return response()->json($request->user());
}
public function logout(Request $request)
{
$request->user()->currentAccessToken()->delete();
return response()->json(['message' => 'Logged out successfully']);
}
}
Step 6: Protect API Endpoints
You can protect your API routes using the auth:sanctum
middleware to ensure only authenticated users access them. Example:
Route::middleware('auth:sanctum')->get('/protected-route', function () {
return response()->json(['message' => 'This is a protected route']);
});
Step 7: Testing the API
- Register a user using the
/register
endpoint. - Login with the credentials to receive an access token.
- Use the access token to make authenticated requests to protected routes by including it in the
Authorization
header:
Authorization: Bearer YOUR_ACCESS_TOKEN
4. Logout by making a POST
request to the /logout
endpoint.
Notes
- Sanctum is suitable for both single-page applications (SPAs) and simple API token use cases.
- For more advanced use cases involving OAuth, consider using Laravel Passport.
This setup provides a basic implementation for Token-Based Authentication using Laravel Sanctum.